
Once seen as intimidating, hard to create, hard to understand blocks of code ChainGPT has taken away the manual redundant labor of copy-pasta and forking and turned the creation of smart contracts into a matter of simply asking good questions.
Quick Start Guide:
1) Go to the ChainGPT AI app: https://app.chaingpt.org/
2) Select “Smart Contract Generator” on the bottom Menu
3) Input a prompt describing what you would like generated
4) Click the “AI Smart Contract Generator”
5) Wait until contract is generated (must remain on web-page)
6) Copy code to clipboard & deploy to GitHub/Remix/ etc…
Step 1:
Navigate to the ChainGPT application interface.
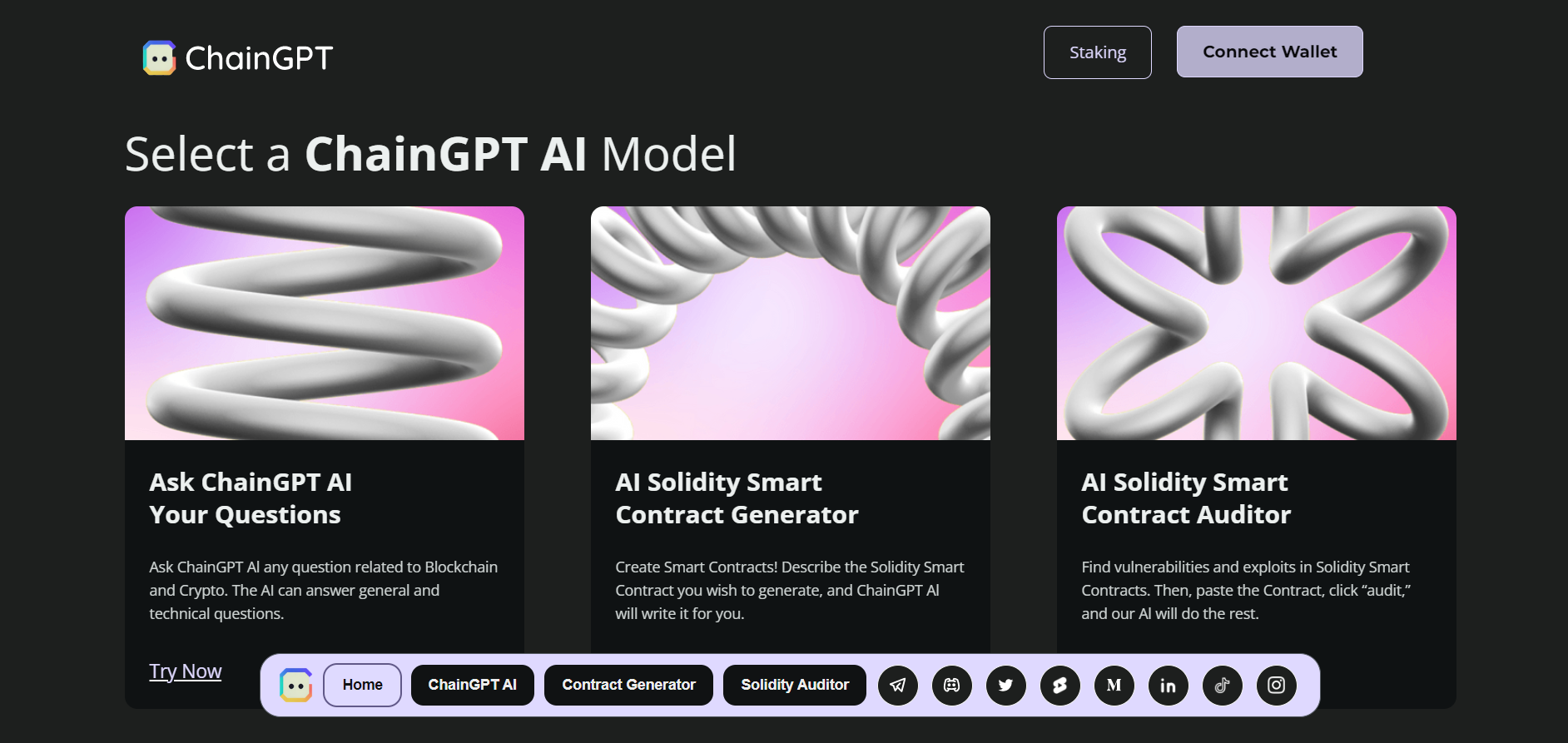
The best way to make sure you are interacting with the official application and avoiding any potential phishing sites is by heading directly to the ChainGPT.org website and clicking on the very top-right of the page where it says “try our Proto-type”.
Alternatively you can hop straight into the app via https://app.chaingpt.org/
Then bookmark it for peace of mind and easy access.
Step 2:
Select the “Contract Generator” option located at the bottom menu bar.

Step 3:
Fill in the text-box with directions and specifications about the kind of contract you want to have created.
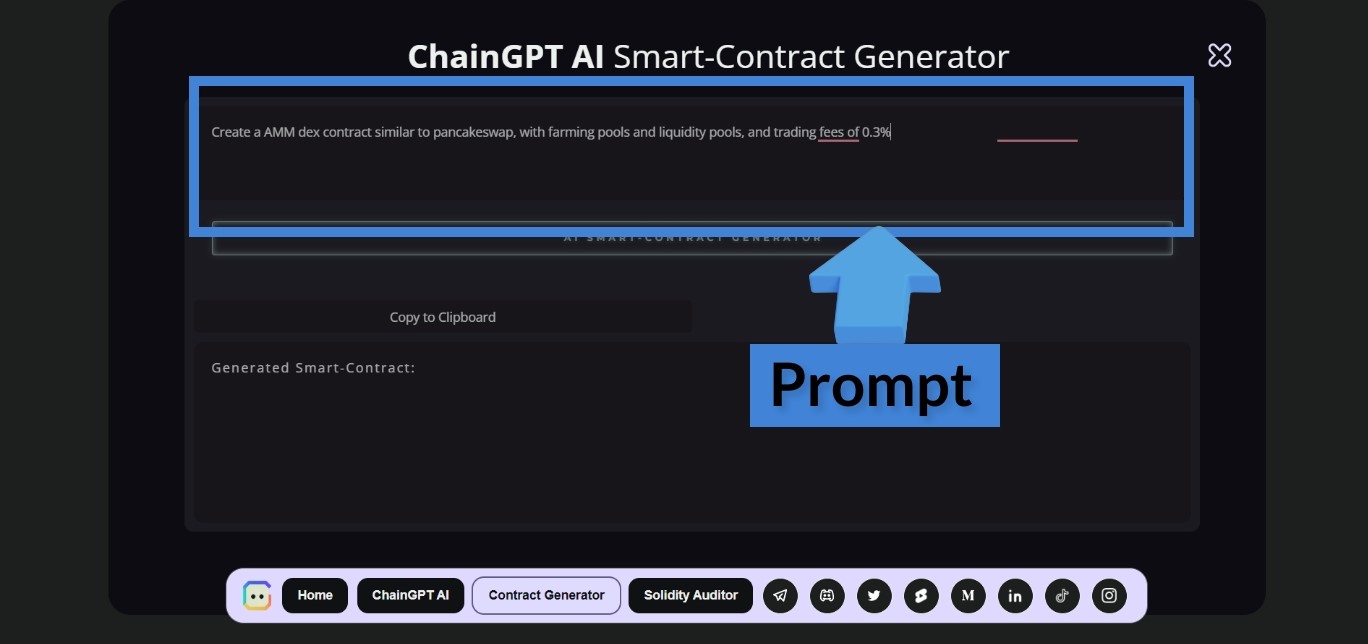
Prompt Examples:
- Token called ChainGPT, symbol CGPT, total supply of 1,000,000,000 tokens.
- Create an NFT collection called “My Master Pony” with 10,000 units in the ERC 1155 standard
- Create a AMM DEX contract similar to Pancakeswap, with farming pools and liquidity pools, and trading fees of 0.3%
Step 4:
Submit your request by clicking the “AI Smart Contract Generator”
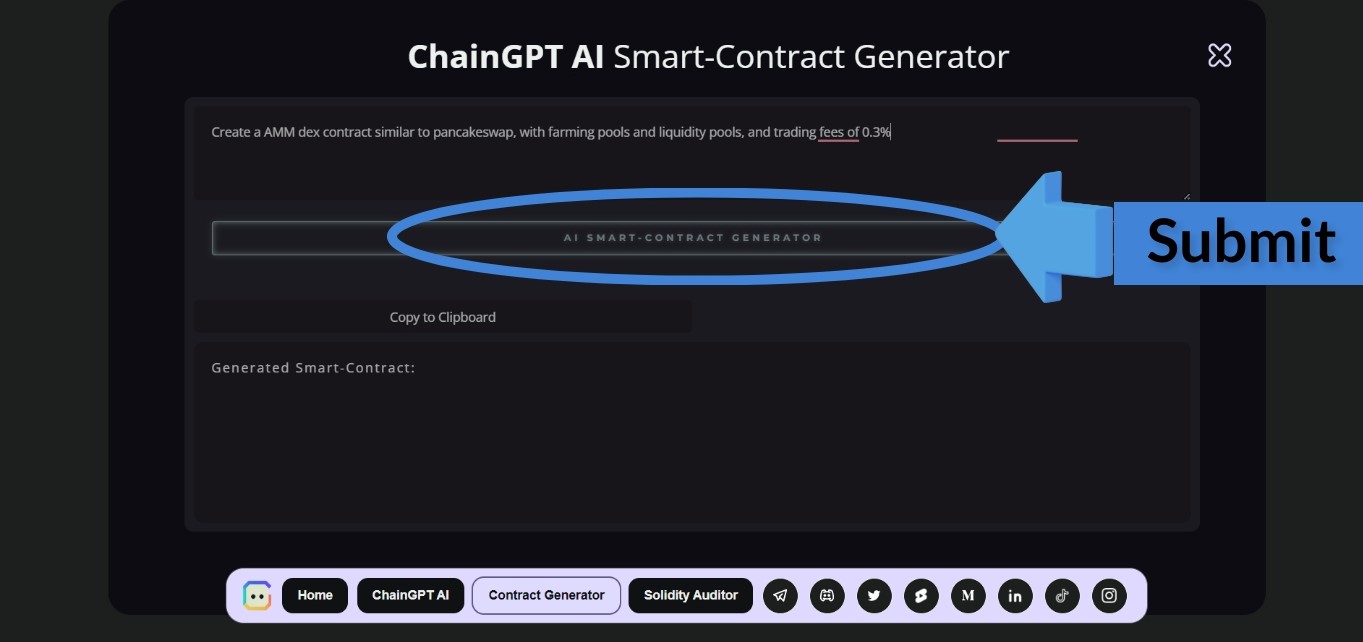
Step 5:
Allow some time for the ChaingGPT generator to process your request. Usually, responses will being to generate within 60 seconds.
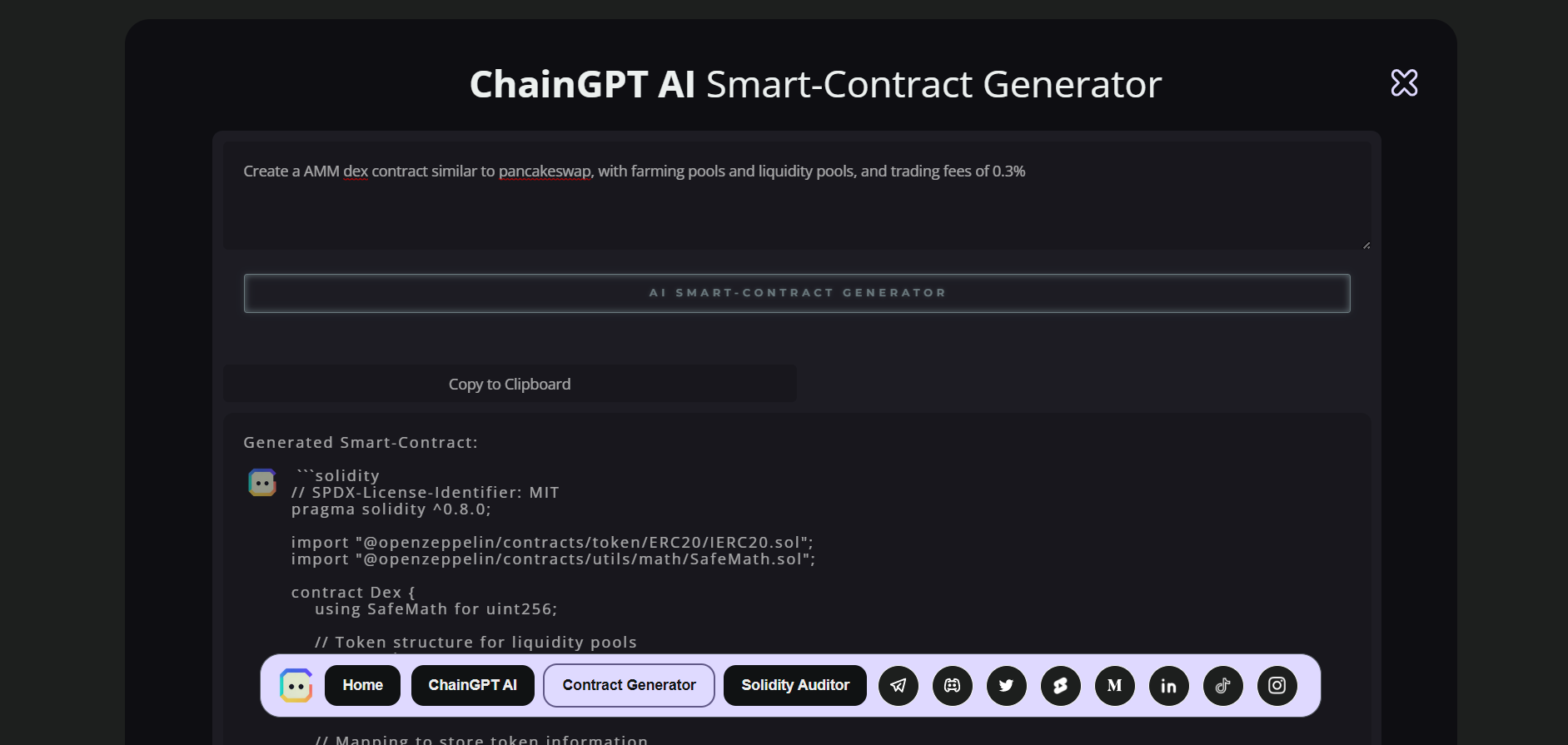
*Note*
The generator only works while you are remain on the webpage. It is ok to leave, but whenever the output process begins, if you switch tabs, ChainGPT will pause until you return.
Step 6:
When ChainGPT completes its response, simply click on the “Copy to Clipboard” button and deploy it to your environment of choice!

*Note*
After the contract is generated, ChainGPT will also provide a basic outline behind the concept of the contract’s concept explaining what libraries and interfaces were used. Be sure to remove this whenever you are deploying!
Example Smart Contract
Prompt:
“ Create a AMM DEX contract similar to Pancakeswap, with farming pools and liquidity pools, and trading fees of 0.3% “
Response:
```solidity
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
import "@openzeppelin/contracts/utils/math/SafeMath.sol";
contract Dex {
using SafeMath for uint256;
// Token structure for liquidity pools
struct Token {
bytes32 ticker;
address tokenAddress;
}
// Mapping to store token information
mapping(bytes32 => Token) public tokens;
// Mapping to store liquidity provider balances
mapping(address => mapping(bytes32 => uint256)) public liquidityProviderBalances;
// Mapping to store token reserves in the liquidity pool
mapping(bytes32 => uint256) public reserves;
// Constants for the AMM
uint256 public constant FEE_DENOMINATOR = 10000;
uint256 public constant FEE_NUMERATOR = 30;
// Events
event AddLiquidity(address indexed user, bytes32 indexed ticker, uint256 amount);
event RemoveLiquidity(address indexed user, bytes32 indexed ticker, uint256 amount);
event Swap(address indexed user, bytes32 indexed fromTicker, bytes32 indexed toTicker, uint256 amountIn, uint256 amountOut);
// Add a new token to the Dex
function addToken(bytes32 ticker, address tokenAddress) external {
tokens[ticker] = Token(ticker, tokenAddress);
}
// Add liquidity to the liquidity pool
function addLiquidity(bytes32 ticker, uint256 amount) external {
IERC20(tokens[ticker].tokenAddress).transferFrom(msg.sender, address(this), amount);
liquidityProviderBalances[msg.sender][ticker] = liquidityProviderBalances[msg.sender][ticker].add(amount);
reserves[ticker] = reserves[ticker].add(amount);
emit AddLiquidity(msg.sender, ticker, amount);
}
// Remove liquidity from the liquidity pool
function removeLiquidity(bytes32 ticker, uint256 amount) external {
require(liquidityProviderBalances[msg.sender][ticker] >= amount, "Not enough balance");
IERC20(tokens[ticker].tokenAddress).transfer(msg.sender, amount);
liquidityProviderBalances[msg.sender][ticker] = liquidityProviderBalances[msg.sender][ticker].sub(amount);
reserves[ticker] = reserves[ticker].sub(amount);
emit RemoveLiquidity(msg.sender, ticker, amount);
}
// Swap tokens using the AMM
function swap(bytes32 fromTicker, bytes32 toTicker, uint256 amountIn) external {
require(reserves[fromTicker] > 0 && reserves[toTicker] > 0, "Insufficient reserves");
uint256 amountOut = getAmountOut(fromTicker, toTicker, amountIn);
IERC20(tokens[fromTicker].tokenAddress).transferFrom(msg.sender, address(this), amountIn);
IERC20(tokens[toTicker].tokenAddress).transfer(msg.sender, amountOut);
reserves[fromTicker] = reserves[fromTicker].add(amountIn);
reserves[toTicker] = reserves[toTicker].sub(amountOut);
emit Swap(msg.sender, fromTicker, toTicker, amountIn, amountOut);
}
// Calculate the amount out for a swap
function getAmountOut(bytes32 fromTicker, bytes32 toTicker, uint256 amountIn) public view returns (uint256) {
uint256 inputReserve = reserves[fromTicker];
uint256 outputReserve = reserves[toTicker];
uint256 amountInWithFee = amountIn.mul(FEE_DENOMINATOR.sub(FEE_NUMERATOR));
uint256 numerator = amountInWithFee.mul(outputReserve);
uint256 denominator = inputReserve.mul(FEE_DENOMINATOR).add(amountInWithFee);
return numerator / denominator;
}
}
```
Ask and you Shall Receive.
Try it out for yourself 👉 https://app.chaingpt.org/#contracts
Resources:
🌐 Website | 📧 Contact | 🤖 Brand | 📃 Whitepaper
Connect with us and Join the community:
Twitter | Telegram | Discord | Instagram | LinkedIn | Youtube | TikTok